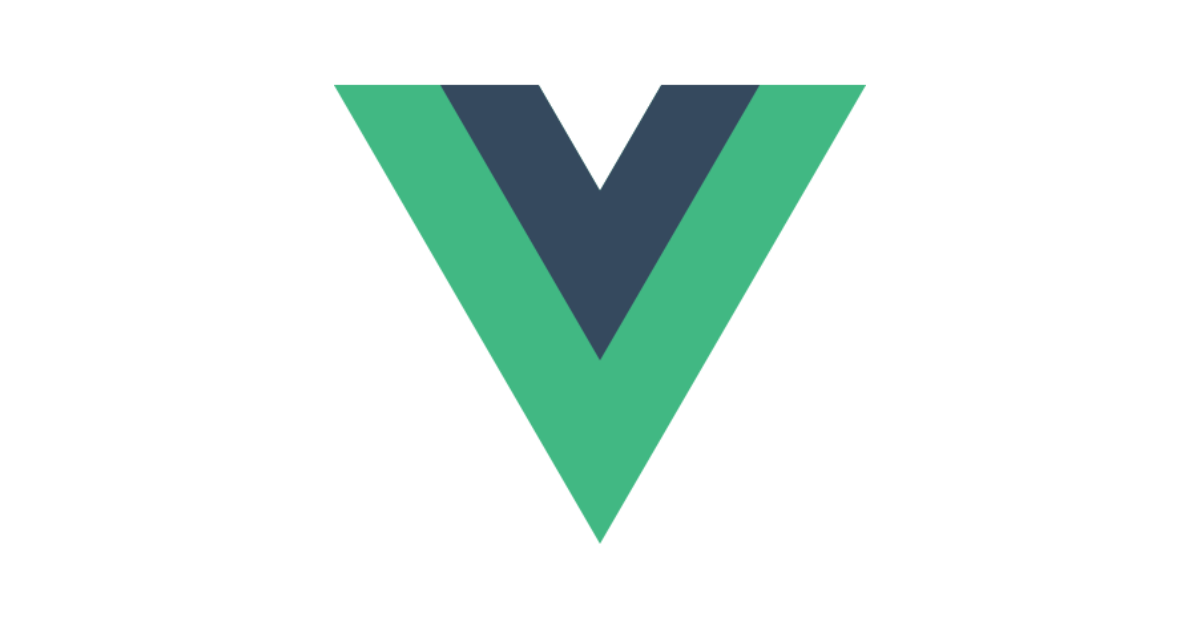
Vue と Jest で Unit test を始めよう
Vue
と Jest
で Unit test
を始めよう
保守性の高いコードが書けていますか?
Vue
のComponent
構成 ~ Unit test
までの流れを書いています。
Unit test
があると、保守しながら長くメンテナンスしていけるコードになるので、
開発の助力になればと思います。
本題の前に
Vue
とは
オープンソースのJavaScript
フレームワークであり、
フロント系では、3大フレームワークと言われ、わかりやすい構文の為人気があります。
Jest
とは
JavaScript
のUnit test
のためのツールです。
わかる事
Atomic Component
のディレクトリ構成 (atoms
)atoms
のComponent
のUnit test
触れないこと
Vue
,Jest
の基本的な構文
Atomic Component
のディレクトリ構成
Unit test
する場合、このようなディレクトリ構成が見通し良いのでおすすめです。
$ tree atoms/ └── MyButton ├── index.test.js └── index.vue
アンチパターンではありませんが 下記の様なディレクトリ構成の場合
MyButton
とmyButton
でファイル名に統一性がないMyButton.story.tsx
などを追加したい場合さらに増える (*storybook
)- 全体の見通しが悪い
$ tree atoms/ ├── myButton.test.js └── MyButton.vue
React
で開発する際も、この構成で開発しています。
Component
のUnit test
Component
の概要
props
で ボタンのテキストをうけとる
click
時は$emit
で、親component
の処理を呼び出す
と なんの変哲もないcomponent
です。
<!--index.vue--> <template> <button @click="$emit('myAction')" > {{ txt }} </button> </template> <script> export const props = { txt: { type: String, required: true }, } export default { props } </script>
Unit test
の概要
ボタンテキストが正しく表示されているか確認
clickイベントの確認
// index.test.js import { shallowMount } from '@vue/test-utils' import MyButton from '@/components/atoms/MyButton' describe('MyButton', () => { const props = { txt: 'MyButtonTxt', } it('ボタンテキストが正しく表示されているか確認', () => { // propsを受け取り shallowMountし テスト対象component生成 const wrapper = shallowMount(MyButton, { propsData: { ...props } }) // {{ txt }}にpropsで受け取った値が表示されているか確認 expect(wrapper.text()).toBe(props.txt) }) it('clickイベントの確認', () => { // propsを受け取り shallowMountし テスト対象component生成 const wrapper = shallowMount(MyButton, { propsData: { ...props } }) expect(wrapper.emitted('myAction')).toBeUndefined() // clickイベント実行 wrapper.trigger('click') // myActionが実行されたか確認 expect(wrapper.emitted('myAction')).toBeTruthy() }) })
まとめ
Atomic Component
の構成にすることのメリット
- テストがしやすい
- ディレクトリの見通しが良い
- 再利用性が高い
Atomic Component
の構成にすることのデメリット
- 1ファイル全て書く方式よりは、ファイル数が増える
1ファイルの行数が長いとUnit test
の際に苦労する場合が多いです。
実際の開発では、機能追加が発生すると思います。
その際バグを含まない用に、Unit test
をしっかり導入していきましょう。